Using PlanetScale OAuth enables your users to connect their accounts to PlanetScale.
Overview
Creating an OAuth application within PlanetScale allows your application to access your users’ PlanetScale accounts.
With PlanetScale OAuth applications, you can choose what access your application needs, and a user will allow (or deny) your application those accesses on their PlanetScale account. The organization that you create the OAuth application in is the "owner" of the application.
Beta
PlanetScale OAuth applications are in beta. Opt-in to the beta's terms of service when you create an OAuth application in your PlanetScale organization's Settings > OAuth applications page.
If you build something you would like to share with us, please email us at education (at) planetscale.com
. We would love to hear about your experience building the application, and we may even feature your application in future blog posts, videos, or social media.
Getting started
1. Creating an OAuth application in PlanetScale
- To create a new OAuth application, log into your organization and click Settings > OAuth applications.
- Create a new OAuth application by clicking Create new application.
- You will need to fill out the following fields:
- Name: A user-friendly name for your OAuth application.
- Domain: The full URL to your application's domain.
- Redirect URI: The full URL PlanetScale should redirect users on completion of the authorization flow, also known as the callback URL. It must have the same domain as the domain above.
- Avatar: An image that represents your OAuth application. (Optional but recommended.)
Note
You will also be agreeing, on behalf of your organization, to prominently display a privacy policy and obtain consent to your organization's terms of use from all users of your products and services.
2. Credentials to copy to your application code
Once you have created your OAuth application in PlanetScale, you will need the following credentials to use the OAuth authorization flow:
- ID: Your OAuth application's ID.
- Client ID: Your OAuth application's client ID.
- Redirect URL: The full URL PlanetScale should redirect users on completion of the authorization flow, also known as the callback URL.
- Client secret: Your OAuth application's client secret, used to exchange access grants for service tokens. (This will only be shown once, make sure to save it!)
Later in this document, we will go through how you use each of these credentials. We recommend saving them as environment variables.
3. OAuth application access scopes
Every OAuth application in PlanetScale will request from its users a specific set of permissions in the users' databases. We call these permissions "access scopes." They are broken into:
- User access
- Organization access
- Database access
- Branch access
Access is scoped to a resource. For example, selecting write_branches
on an organization allows you to write branches across all databases in organizations the user gives permission to, while write_branches
on a database enables you to only write branches in databases the user gives permission to.
The API reference for each endpoint will say what scope is needed.
In this step, select the access scopes you think your application will need on a user's account and click the Save access scopes button.
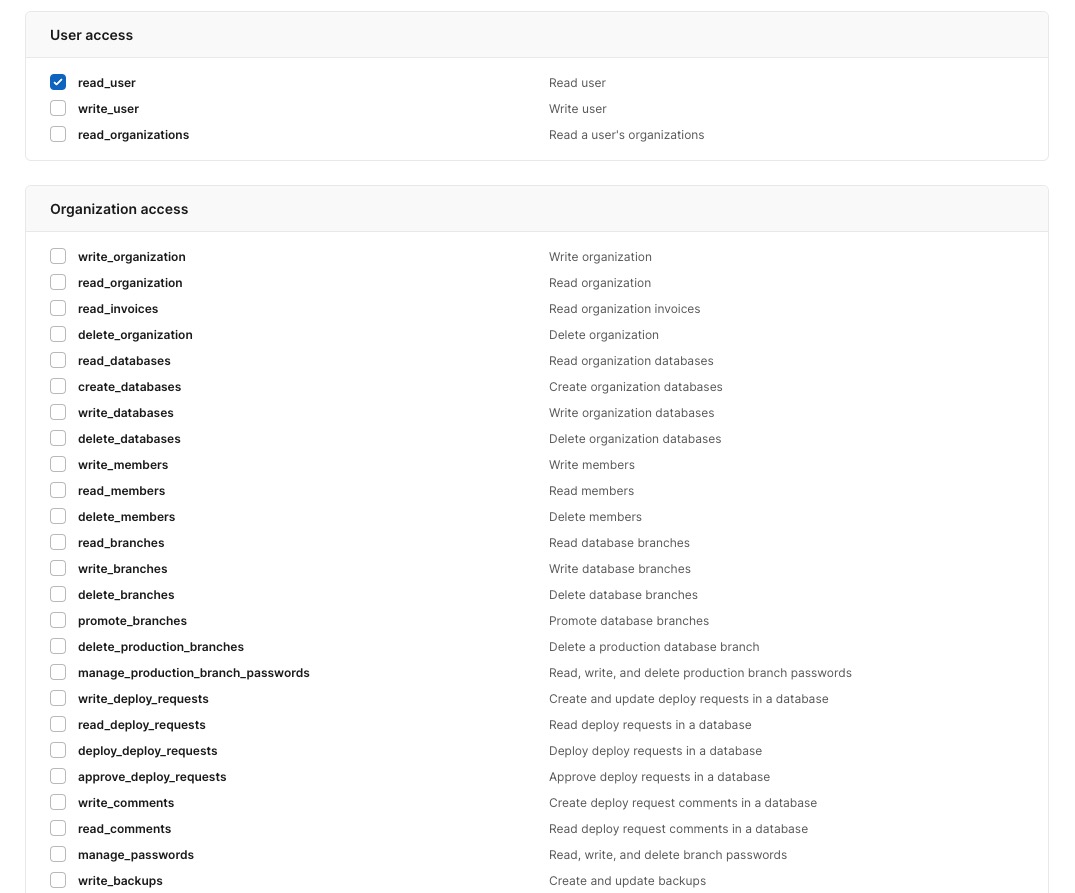
This is only a partial list of the OAuth access scopes. For a full list of scopes, see the OAuth access scopes documentation.
OAuth Authorization Flow
PlanetScale's OAuth implementation supports the Authorization Code grant type. The following diagram walks through the flow.
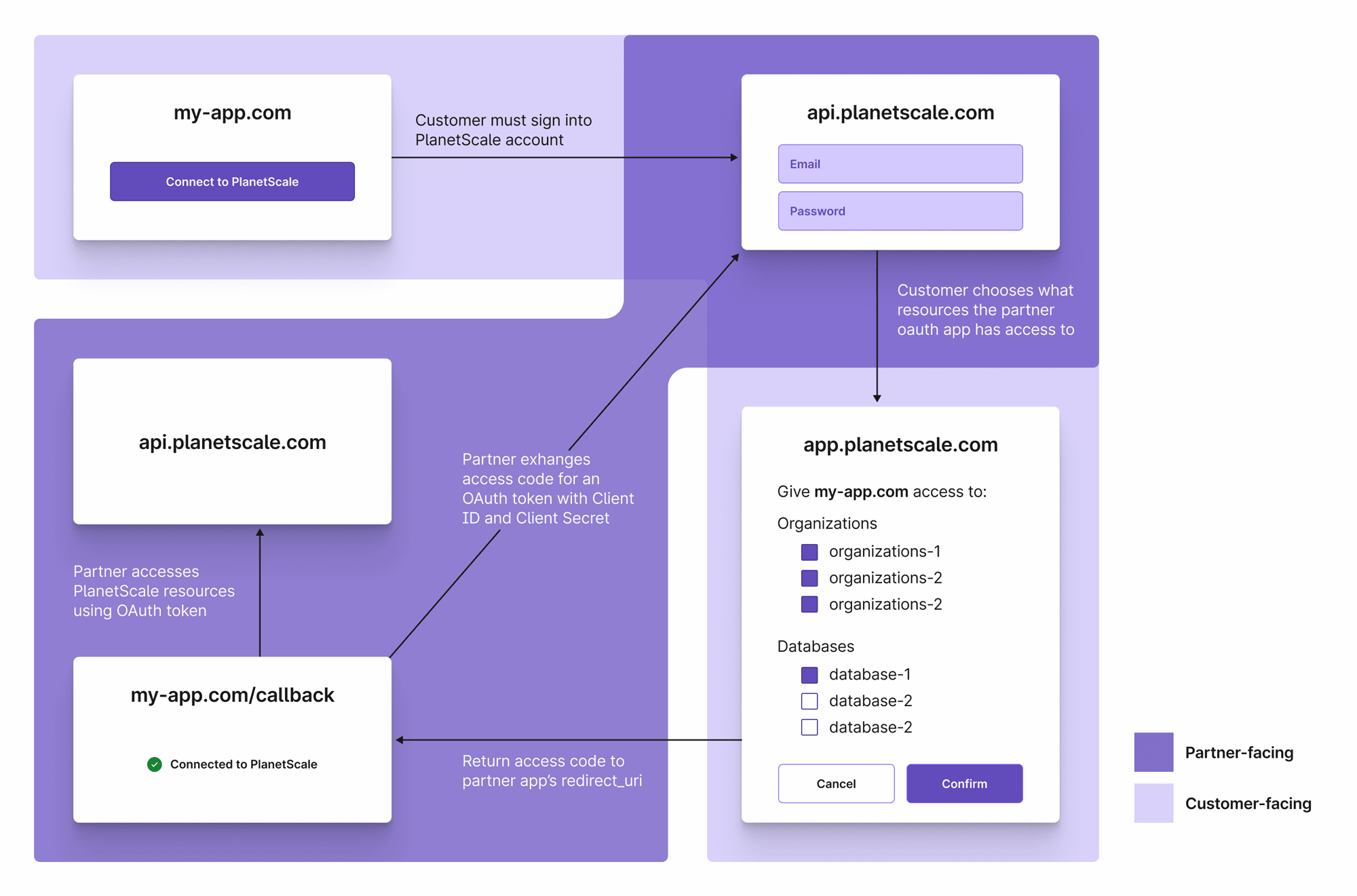
OAuth authorization flow diagram
0. Prerequisites
You must have created a service token in your OAuth application's organization.
Copy and paste the ID and service token into your code, where the rest of your important credentials are stored.
A service token is needed to use the PlanetScale API to create OAuth tokens as a part of the OAuth authorization flow. You will need the following organization-level accesses on the service token in order to complete the flow: read_oauth_applications
, write_oauth_tokens
, read_oauth_tokens
.
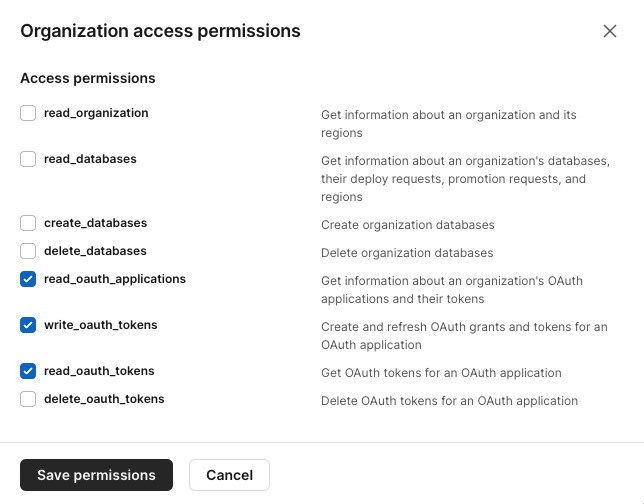
1. User authorizes your OAuth application on their account
Your application should direct your users to the PlanetScale authorization page (see URL below) so that they can grant your application access to their PlanetScale account:
http://app.planetscale.com/oauth/authorize?client_id=CLIENT_ID&redirect_uri=REDIRECT_URI&state=STATE
Query parameters:
Name | Type | Description |
---|---|---|
client_id | string | Your OAuth application's client id |
redirect_uri | string | The full URL PlanetScale should redirect users on completion of the authorization flow, also known as the callback URL. |
state (optional) | string | You may also optionally pass a state parameter, which exists to prevent third-party attacks. Pass a random string, and PlanetScale will return it in step 2. Compare to ensure the request came from your application. |
2. The authorization code returns to your application
Upon authorization, PlanetScale will redirect the user to your redirect_uri
with an authorization code in the query parameters. The authorization code is only good for one use. It will look like the following URL:
https://my-redirect-uri.com?code=AUTHORIZATION_CODE&state=STATE
Query parameters:
Name | Type | Description |
---|---|---|
code | string | An authorization code to be exchanged for an access token. |
state (if you provided one) | string | Compare with the original state parameter to ensure they match. Abort the process if they do not because the request may have come from a third party. |
3a. Exchange authorization code for an OAuth token
Your application can now exchange the authorization code for an access token.
POST
https://api.planetscale.com/v1/organizations/:organization_name/oauth-applications/:application_id/token?client_id=CLIENT_ID&client_secret=CLIENT_SECRET&code=CODE&grant_type=authorization_code&redirect_uri=REDIRECT_URI
The POST
request will need the following:
Headers:
Authorization: <SERVICE_TOKEN_ID>:<SERVICE_TOKEN>
Path parameters:
Name | Type | Description |
---|---|---|
organization_name | string | Your organization name. |
application_id | string | Your OAuth application's ID (12 character long ID). |
Query parameters:
Name | Type | Description |
---|---|---|
client_id | string | Your OAuth application's client ID. |
client_secret | string | Your OAuth application's client secret. |
code | string | The code located in the query parameters of the previous step. |
grant_type | string | Set to authorization_code . |
redirect_uri | string | The full URL PlanetScale should redirect users on completion of the authorization flow, also known as the callback URL. |
The response will look similar to the following:
{
"id": "cv4d3zi653gv",
"type": "ServiceToken",
"display_name": "My OAuth App's Service Token cv4d3zi653gv",
"avatar_url": "https://my-oauth-app.com/avatar.png",
"created_at": "2022-08-01T20:19:41.886Z",
"updated_at": "2022-08-01T20:19:41.886Z",
"expires_at": "2022-09-01T20:19:41.887Z",
"last_used_at": null,
"name": "my-oauth-app",
"token": "pscale_tkn_O4KbFjH97uOz2bLWJtQXjYgDsqkGgC8bNNlrzgo6YUY",
"plain_text_refresh_token": "pscale_tkn_W_zjmZ1a14sczj15bxJdsW_kiv063OrHG4CBh0IXR9M",
"actor_id": "r80q66antldo",
"actor_display_name": "[email protected]",
"actor_type": "User",
"service_token_accesses": [...]
}
The three most essential credentials in the response are:
id
: Your access token's ID.token
: Your access token.plain_text_refresh_token
: A refresh token that can refresh your access token when it expires. See step 3b for more info.
You will need both the id
and token
to make API calls on behalf of the user.
3b. Refreshing an OAuth token
When an OAuth token expires, you can refresh it:
POST
https://api.planetscale.com/v1/organizations/:organization_name/oauth-applications/:application_id/token?client_id=CLIENT_ID&client_secret=CLIENT_SECRET&grant_type=refresh_token&refresh_token=REFRESH_TOKEN
The POST
request will need the following:
Headers:
Authorization: <SERVICE_TOKEN_ID>:<SERVICE_TOKEN>
Path parameters:
Name | Type | Description |
---|---|---|
organization_name | string | Your organization name. |
application_id | string | Your OAuth application's ID. |
Query parameters:
Name | Type | Description |
---|---|---|
client_id | string | Your OAuth application's client ID. |
client_secret | string | Your OAuth application's client secret. |
refresh_token | string | The refresh token sent in the initial token response in step 3a. |
grant_type | string | Set to refresh_token . |
The response will look similar to the response in 3a.
4. Using the OAuth token
Now your application can make requests to the PlanetScale API on behalf of the user. To make requests to the API, add the id
and token
in the Authorization
header in your HTTP API request using the following format:
Authorization: <OAUTH_TOKEN_ID>:<OAUTH_TOKEN>
Now your application can make requests to the PlanetScale API on behalf of the user. To make requests to the API, add the id
and token
in the Authorization
header in your HTTP API request using the following format:
Authorization: <OAUTH_TOKEN_ID>:<OAUTH_TOKEN>